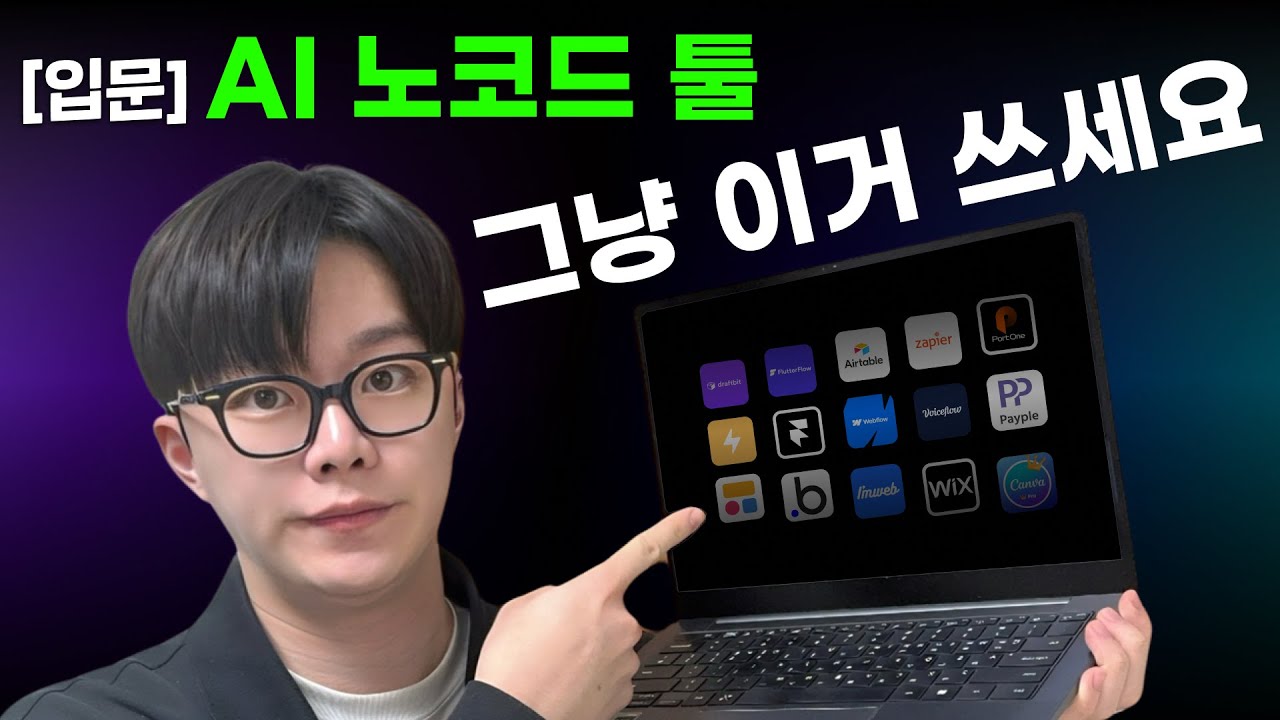
자동화 툴 - 공부해보기
https://www.youtube.com/watch?v=ywH7JIK34Tg
- yeji KimY
-- Fetch related textbook pages for a specific slide
SELECT tp.page_number, tp.content, t.textbook_title
FROM slide_textbook_mapping stm
JOIN textbook_pages tp ON stm.page_id = tp.page_id
JOIN textbooks t ON tp.textbook_id = t.textbook_id
WHERE stm.slide_id = ?;
-- Fetch related test questions for a specific slide
SELECT tq.question_text, tq.exam_year, tq.exam_type
FROM slide_question_mapping sqm
JOIN test_questions tq ON sqm.question_id = tq.question_id
WHERE sqm.slide_id = ?;
-- Fetch related lecture slides for a test question
SELECT ls.slide_title, ls.slide_content
FROM slide_question_mapping sqm
JOIN lecture_slides ls ON sqm.slide_id = ls.slide_id
WHERE sqm.question_id = ?;
-- Fetch related textbook pages for a test question
SELECT tp.page_number, tp.content, t.textbook_title
FROM question_textbook_mapping qtm
JOIN textbook_pages tp ON qtm.page_id = tp.page_id
JOIN textbooks t ON tp.textbook_id = t.textbook_id
WHERE qtm.question_id = ?;
-- Fetch all lecture notes for a professor
SELECT ln.title, ln.version, ln.upload_date
FROM lecture_notes ln
WHERE ln.professor_id = ?;
-- Fetch all test questions from a professor
SELECT tq.question_text, tq.exam_year, tq.exam_type
FROM test_questions tq
WHERE tq.professor_id = ?;
-- Insert a new version
INSERT INTO lecture_note_versions (lecture_note_id, version_number, uploaded_at, file_path, notes)
VALUES (?, ?, NOW(), ?, ?);
-- Update the lecture note to reflect the latest version
UPDATE lecture_notes
SET version = ?, upload_date = NOW()
WHERE lecture_note_id = ?;